What are hooks?
According to the React documentation, hooks provides us a way to use state and other features of React without writing a class component. Hooks let you use the class component’s lifecycle features inside a function component.
Initially, when I started learning React, I had no idea about hooks. I followed the class-oriented component approach and heavily used it in my projects. Later, when I got introduced to the concept of hooks, I was amazed by its simplicity of it. I started learning everything I could about hook from the official React documentation. Hooks improved my developer experience of writing components in React.
I would like to suggest you to have some understanding of class components before reading the entire article.
Why hooks?
The following are some advantages of using hooks:
• Hooks make it easier to write components.
• Less code compared to class components.
• Makes code cleaner and easier to understand.
Rules
Though the concept of hooks is amazing, you have to understand the following rules:
• Hooks can be used only inside a function component. We cannot use hooks inside a class component.
• Hooks cannot be used inside loops, conditions and nested functions.
React has several built-in hooks as well as it allows us to create our own custom hooks. Following are some built in hooks:
• useState
• useEffect
• useRef
• useContext
Also, there are other advanced hooks available such as useReducer, useCallback and many more. In this article, we are going to study in depth about useState hook.
useState
As the name says, useState hook is used to implement class like state inside a functional component. The useState hook is a part of a standard React library. You have to import the hook before implementing it inside a function.
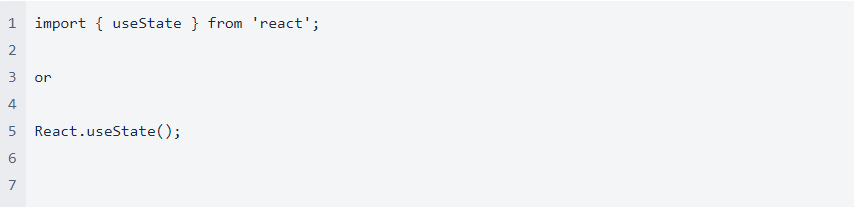
The useState returns two things – first is a state variable that holds the actual data, the second is a function which can be used to manipulate the state. Let us discuss this with an example mentioned below:
Whenever we add a new item inside a cart on any shopping website, it displays the count of total items available in a cart. We can implement this functionality using useState hook.
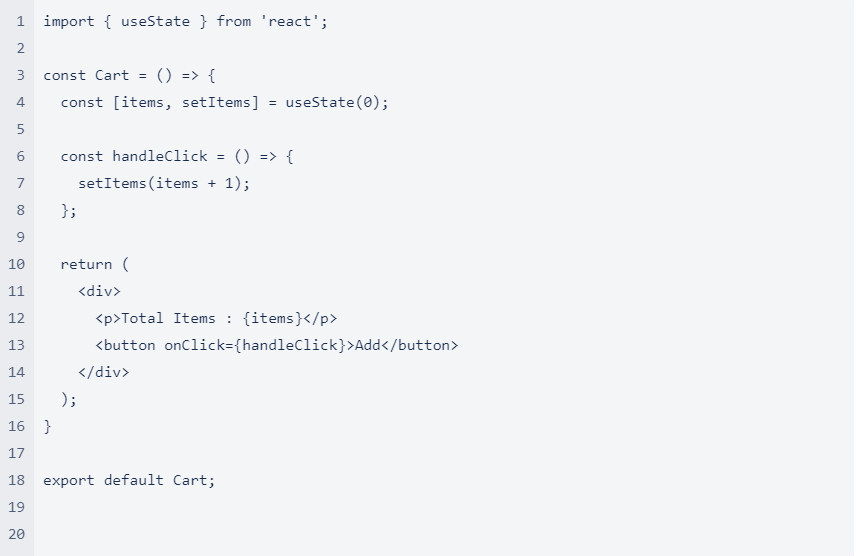
In the above example, items is a state variable that holds the actual data and setItems is a function which is used to modify the data present inside the items variable. We have created a function called handleClick that increments state by one whenever the button is clicked.
You can pass a default value for the state variable as a parameter to useState. In this example, by default items variable contains 0. After button click event, handleClick function is invoked which increments value by 1. After the function call the value of items becomes 1.
You can pass string, array as well as an object to the useState. The useState hook can also be used to store data returned by an API.
Standard Practice
In the above example, we have directly modified the state by calling setItems(items + 1). Instead, you should use the previous value as a function argument and then return a new value.
